React is a JavaScript library used for building interactive user interfaces (UI) for web applications. It has gained immense popularity among developers due to its efficient rendering and component-based architecture. Consequently, there has been a significant rise in the number of people taking React Native Training Courses.
Key Features of React:
- Component-Based Architecture: React enables the creation of reusable user interface components, simplifying the management and upkeep of complex UI structures.
- Virtual DOM: To speed up rendering and improve performance, React makes use of a virtual DOM, a thin duplicate of the real DOM.
- Declarative Syntax: React uses declarative syntax, allowing developers to specify how the user interface (UI) should appear depending on its current state, with rendering and updates being handled by React automatically.
- One-Way Data Binding: React encourages one-way data binding, in which data travels in a single path, making application performance and debugging easier.
Let's explore the key features, benefits, and use cases of React.
Why Choose React?
React is a popular option among developers because of its many benefits. The following are some strong arguments for the widespread usage of React:
Efficiency
Only the relevant components are effectively managed and updated by React's virtual DOM, which leads to quicker rendering and better performance. React enhances overall web application performance by reducing DOM manipulation.
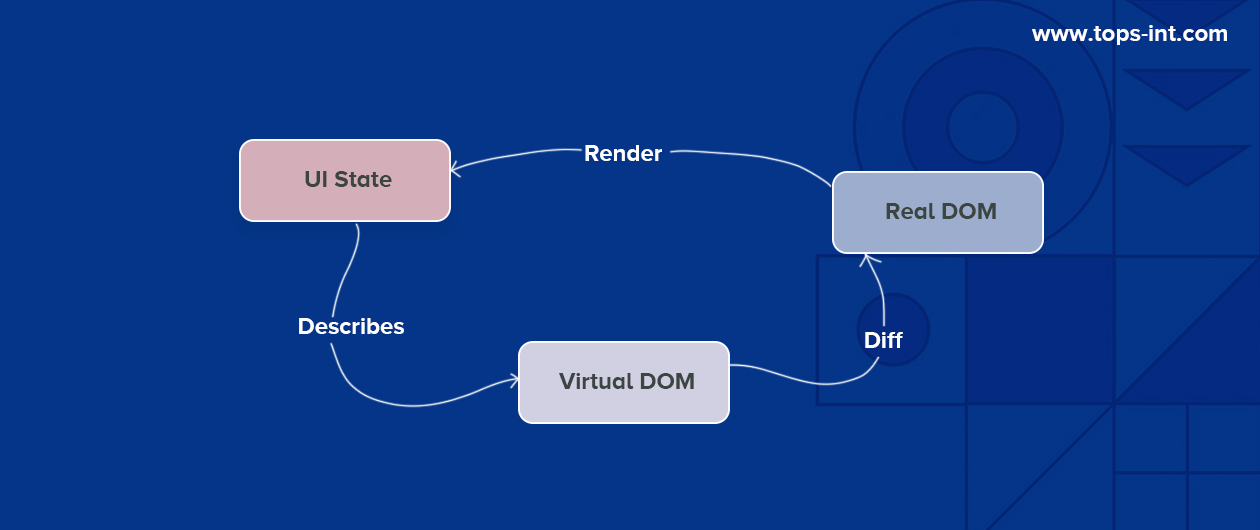
Declarative grammar
React's declarative grammar enables programmers to specify the UI's appearance depending on various conditions. As a result, developers just need to concentrate on the intended output rather than the manual DOM manipulations, which makes code simpler to comprehend and maintain.
Unidirectional Data Flow
Data travels in a single way inside the component hierarchy while using React because of its unidirectional data flow. As a result, debugging is made simpler, and it is simpler to comprehend how data changes spread across the programme.
Large Ecosystem
React has a robust community and a large ecosystem. This indicates that there are many frameworks, resources, and tools available that enhance React's functionality and speed up and streamline development.
Here are some official resources that highlight the large ecosystem of React:
React Documentation: The official React documentation is a comprehensive resource for understanding the library and its features.
React GitHub Repository: The official GitHub repository of React where you can find the source code, contribute to the project, and report issues.
React Community: This page provides a list of community resources for React developers, including forums, chat rooms, and community support.
React Blog: The official blog of React where updates, new features, and community highlights are posted.
React Native: The official website for React Native, a framework for building native apps using React.
React Router: The standard routing library for React, which lets you create dynamic routes in your applications.
React Redux: Official React bindings for Redux, a predictable state container for JavaScript apps.
React Native
JavaScript programmers may create native mobile apps for the iOS and Android platforms using the React Native framework, which is built on React. By using React Native, developers can construct cross-platform mobile applications faster and easier than before.
SEO-Friendly
Server-side rendering (SSR) features offered by React make it easier for search engines to index and crawl the content of online apps. Search engine optimisation (SEO) is improved, and websites built using React are easier to find.
Features Of React
ReactJS offers several intriguing features that make it a popular choice among developers.
JSX
JSX (JavaScript XML) is a syntax extension used by React to combine HTML-like syntax with JavaScript code. It allows developers to embed HTML code inside JavaScript, making it easier to understand and maintain. JSX is transformed into standard JavaScript objects using preprocessors like Babel, enhancing code readability and boosting application performance.
Virtual DOM
React utilises a virtual DOM, which is a lightweight representation of the actual DOM. The virtual DOM is a tree-like structure that lists elements, their attributes, and content as objects and properties. React render function creates this virtual DOM representation of React components. When the underlying data changes, React calculates the differences between the previous and new virtual DOM, and updates the actual DOM with only the necessary changes.
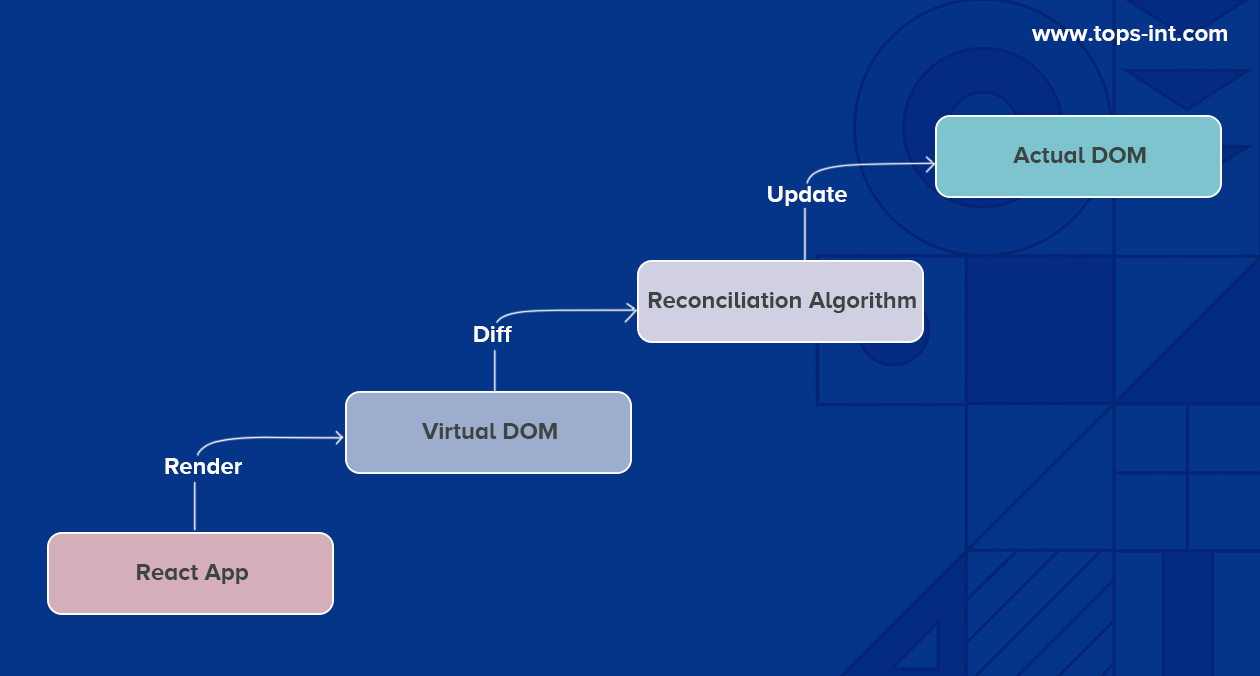
Testability
React's component-based architecture and the ability to manipulate component state make React applications highly testable. Developers can easily manipulate the state of components and observe the output and triggered actions, events, and functions. This simplifies testing and debugging processes, ensuring the reliability and stability of React applications.
Server-Side Rendering (SSR)
React supports server-side rendering, allowing developers to pre-render the initial state of React components on the server. With SSR, the server sends HTML of the page to the browser, which can start rendering immediately without waiting for all JavaScript to load and execute. This improves the perceived performance and user experience, as the webpage becomes visible even before the client-side rendering completes.
One-Way Data Binding
React follows a unidirectional data flow or one-way data binding. Data flows from parent components to child components, preventing unexpected changes and improving control over the application's state. The application's state is contained in specific stores, making components loosely coupled and enhancing flexibility and efficiency.
The popularity of ReactJS continues to grow among developers due to its component-based architecture, performance optimisation through virtual DOM, strong community support, and its ability to handle complex web applications efficiently.
Components In React
What are components ?
Components in React are self-contained building blocks that encapsulate a part of the user interface (UI) and its functionality. They allow developers to break down the UI into smaller, reusable pieces, making the code more modular, maintainable, and easy to understand. React components can be class-based or function-based.
Here's an example of creating React components using code:
Class-Based Component:
In the above code, we define a class-based component called Greeting. The component extends the React.Component class and overrides the render method to define the component's output. In this case, the render method returns anelement that displays a greeting with the value of the name prop passed to the component.
Function-Based Component:
In the function-based component, we define a JavaScript function called Greeting. The function takes props as an argument and returns the JSX representation of the component's output. Similar to the class-based component, we pass the name prop to display a personalised greeting.
To use these components in another component or application, we can import and render them as follows:
In the above code, the Greeting component is imported and used within the App component. We pass the name prop with different values to create multiple instances of the Greeting component, each displaying a personalised greeting.
React utilises a virtual representation of the DOM, known as the Virtual DOM, to optimise the process of updating and rendering UI components. This technique enhances performance and improves the overall efficiency of React applications.
How does the Virtual DOM Work in React?
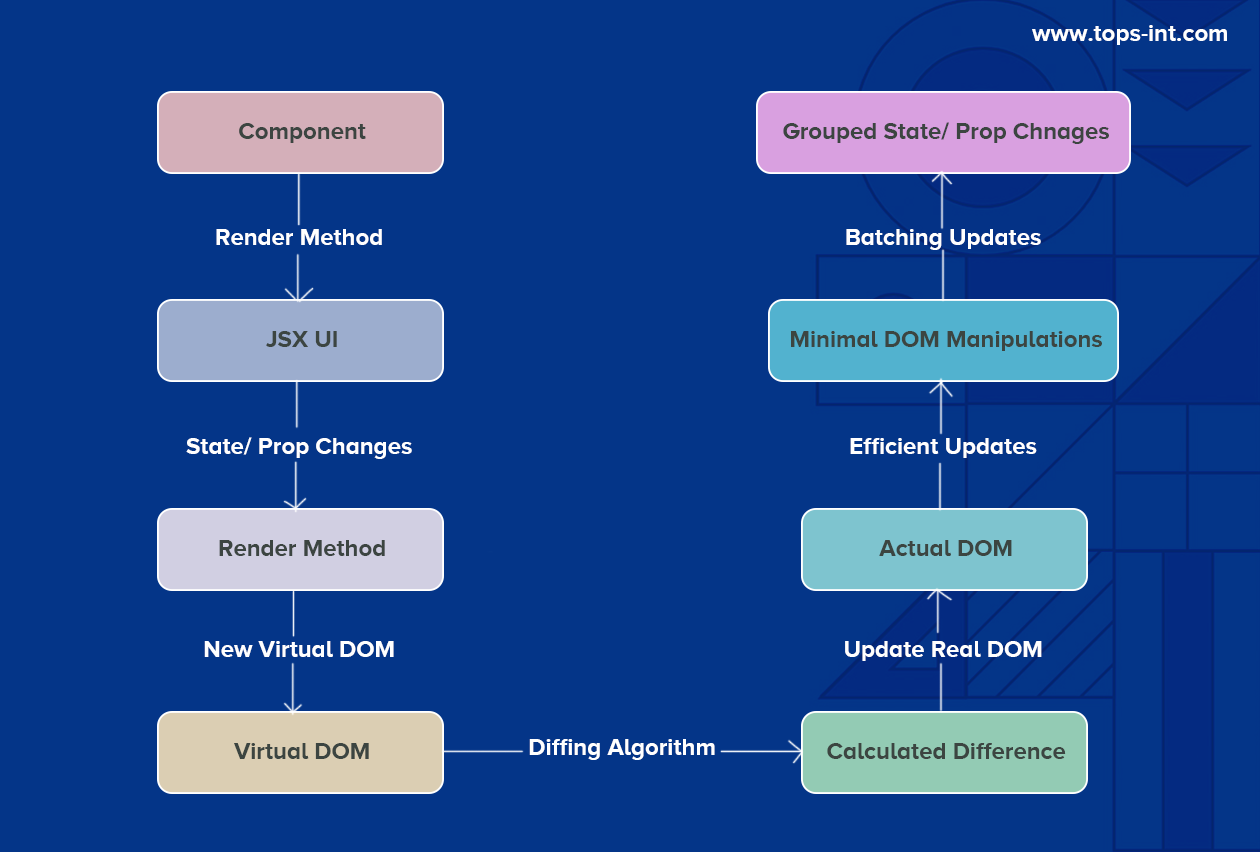
- Component Rendering: React components have a render() method that returns a JSX representation of the component's UI. When a component's state or props change, the render() method is called again to generate a new Virtual DOM representation.
- Virtual DOM Creation: React creates a lightweight copy of the actual DOM by constructing a tree-like structure that mirrors the structure of the UI. This Virtual DOM representation is made up of objects and properties that correspond to elements, attributes, and content.
- Diffing Algorithm: React utilises a powerful diffing algorithm to compare the new Virtual DOM with the previous one. It identifies differences and calculates the minimal set of changes required to update the actual DOM efficiently.
- Calculating Differences: During the diffing process, React compares each element in the new Virtual DOM with its corresponding element in the previous Virtual DOM. It examines attributes, content, and the presence of new or removed elements to determine the necessary updates.
- Updating the Real DOM: Once the differences are calculated, React applies the necessary changes to the actual DOM. Instead of updating the entire DOM tree, React selectively updates only the affected elements, optimising the rendering process and reducing unnecessary manipulations.
- Efficient Updates: By leveraging the Virtual DOM and the diffing algorithm, React minimises the number of DOM manipulations required. It calculates and applies only the changes necessary to synchronise the actual DOM with the new state or props of the components. This approach significantly improves performance and avoids unnecessary re-rendering of unchanged elements.
- Batching Updates: React utilises a technique called "batching" to optimise updates. It groups multiple state or prop changes into a single update, reducing the number of re-renders and enhancing performance.
Code Example:
Here's an example showcasing the usage of the Virtual DOM in React:
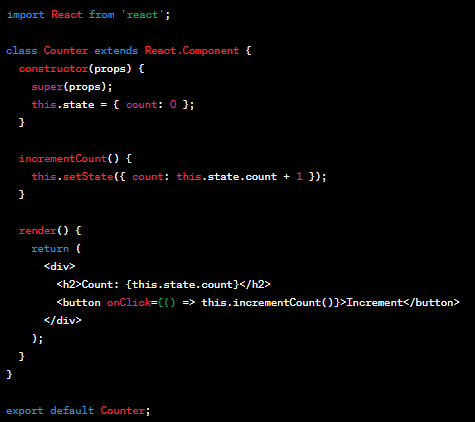
In the above code, the Counter component maintains a count value in its state. When the button is clicked, the incrementCount() function is called, updating the state and triggering a re-render of the component and its corresponding Virtual DOM.
By leveraging the Virtual DOM, React efficiently updates only the necessary elements to reflect the changes in the UI, resulting in an optimised rendering process.
How does JSX function and what is it?
React uses the syntactic extension JSX (JavaScript XML), which combines JavaScript code with HTML-like syntax. It makes it simpler to construct and work with the UI components in React by enabling developers to write HTML-like code directly inside JavaScript.
How JSX Works:
- Syntax Extension: JSX extends the ECMAScript syntax, allowing XML/HTML-like text to coexist with JavaScript code. It enables developers to embed HTML code directly into JavaScript functions or classes.
- Babel Transformation: JSX code is not directly understood by browsers. To make JSX compatible with JavaScript, a tool like Babel is used. Babel transforms JSX syntax into regular JavaScript function calls, which can be understood by browsers and executed.
- Compilation Process: During the compilation process, JSX is transformed into JavaScript objects known as React elements. These elements describe the structure and properties of UI components. React then uses these elements to create and update the Virtual DOM.
Code Example:
Here's an example showcasing how JSX is used in React components:
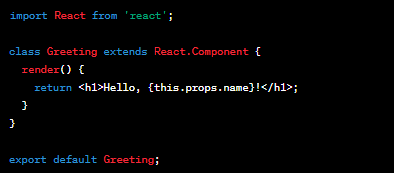
In the above code, we define a class-based component called Greeting. Within the render() method, we use JSX syntax to define the structure of the component's output.
React internally transforms this JSX code into JavaScript function calls, equivalent to:

The transformed code creates a React element with the specified type (h1), no additional properties (null), and the content (Hello, {this.props.name}!) as its child.
During the rendering process, React utilises these React elements to construct the Virtual DOM representation and efficiently update the actual DOM based on the component's state and props.
State and Props and how to use them ?
In React, both state and props are used to manage and pass data to components, but they serve different purposes. Let's explore the concepts of state and props and see how to use them in React code:
State:
- State is a built-in feature in React that allows components to manage and store their internal data.
- It represents the mutable data that can change over time, affecting the component's behaviour and rendering.
- State is initialised and managed within a component using the setState() method.
- Only class components can have state, and it is stored as an object property within the component's state property.
- Changes to the state trigger a re-rendering of the component, updating the UI to reflect the new state.
Here's an example of using state in a React component:
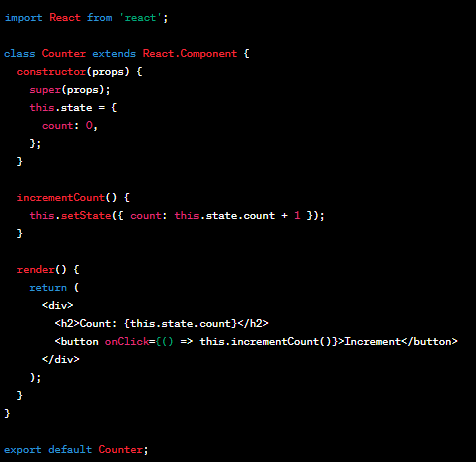
In the above code, the Counter component maintains a count state property within its state object. The initial value is set to 0 in the component's constructor. The incrementCount() method updates the count state using setState() whenever the button is clicked.
Props:
- Props (short for properties) are used to pass data from a parent component to its child component(s).
- Props are read-only and cannot be modified by the child component.
- They are passed as attributes to the child component in the parent's JSX.
- Props enable parent components to control the behaviour and data of child components, making them reusable and customisable.
Here's an example of using Props in React:
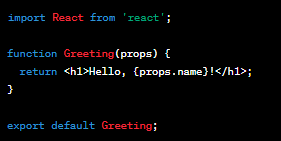
In the above code, the Greeting component receives the name prop and displays a personalised greeting using that value.
To use the components with state and props in another component or application, you can import and render them as follows:
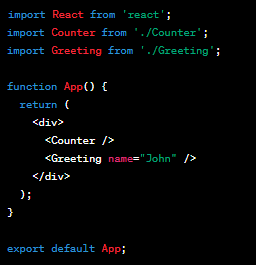
In the App component, we render both the Counter component (with its internal state) and the Greeting component (with a prop). This demonstrates how state and props can be used together in a React application.
What are React Hooks? What Are Their Types?
React 16.8 included the React Hooks feature, which enables functional components to leverage state and other React capabilities without having to create class components. Hooks provide functional components a more succinct and adaptable approach to handle state and lifespan.
There are several built-in React Hooks, each serving a specific purpose. Let's explore some commonly used React Hooks and see how they can be implemented in code:
1. useState:
- The useState hook allows functional components to manage state.
- The state variable can be of any data type, such as a string, number, array, or object.
- To update the state, the function returned by useState is called.
Here's an example of using the useState hook:
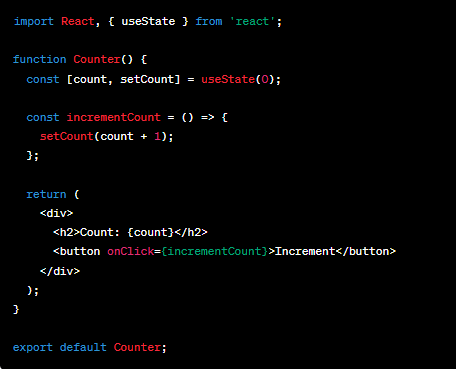
In the above code, the useState hook is used to declare the count state variable and the setCount function to update it. The initial value of count is set to 0. The incrementCount function updates the state by calling setCount with the new value.
2. useEffect:
- The useEffect hook allows performing side effects in functional components, such as fetching data, subscribing to events, or manipulating the DOM.
- It is similar to the lifecycle methods in class components, like componentDidMount, componentDidUpdate, and componentWillUnmount.
- The useEffect hook takes a callback function as its first argument and an optional dependency array as its second argument.
- The callback function is executed after every render or when the dependencies change.
Here's an example of using the useEffect hook:
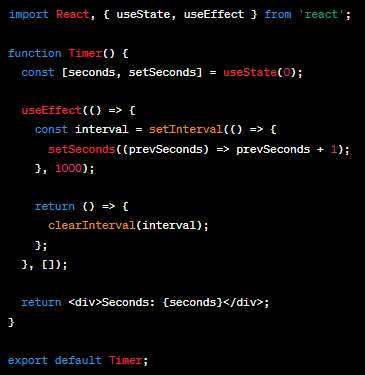
In the above code, the useEffect hook is used to start a timer that increments the seconds state variable every second. The cleanup function returned by useEffect is used to clear the interval when the component unmounts.
3. useContext:
- The useContext hook allows accessing the value of a context in functional components.
- It is used to consume data provided by a context provider higher up in the component tree.
- The useContext hook takes a context object as its argument and returns the current context value.
Here's an example of using the useContext hook:
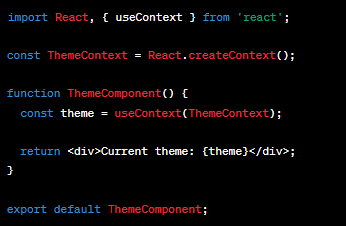
In the above code, the useContext hook is used to access the value of the ThemeContext. The ThemeComponent can consume and display the current theme value provided by a ThemeProvider higher up in the component tree.
These are just a few examples of React Hooks. React also provides other hooks like useReducer, useCallback, and useMemo, among others, that offer additional functionalities and flexibility in functional components.
By utilising React Hooks, developers can write more concise and expressive code in functional components, effectively managing state, side effects, and other React features.
Conclusion
The adoption of React in India is witnessing significant growth as businesses and developers recognize its power and efficiency in building modern web applications. To secure a bright future in the field of web development, enrolling in a React course is highly recommended. It equips individuals with the necessary skills and knowledge to excel in the industry.
TOPS Technologies stands out as one of the best institutes for React training. With its comprehensive curriculum, experienced trainers, and hands-on approach, TOPS Technologies provides aspiring developers with the expertise and confidence to leverage React effectively.
Investing in React education at TOPS Technologies can pave the way for exciting career opportunities in the dynamic world of web development. TOPS Technologies provides over 50 plus courses like
CSS Training Courses, Mobile development and a lot more!
FAQs
How does React differ from other JavaScript frameworks?
React differs from traditional JavaScript frameworks by utilising a virtual DOM, which allows for efficient rendering and updates. Additionally, React follows a component-based architecture, promoting reusable and modular UI development.
What are React hooks, and why are they important?
React hooks are functions introduced in React 16.8 that allow developers to use state and other React features without writing a class. They provide a more concise and intuitive way of managing state and side effects in functional components, making the code easier to read and maintain.
What is the significance of JSX in React?
JSX is an extension to JavaScript that enables developers to write HTML- like code within their JavaScript files. It allows for a more declarative and intuitive way of defining components and their structure, making the code more readable and maintainable.
What are the prerequisites for React Native Training?
To get the most out of
React Native Training, a basic understanding of JavaScript and React concepts is recommended. Familiarity with HTML, CSS, and mobile app development concepts will also be advantageous. However, some training programs cater to beginners and provide the necessary foundation.
Where can I find a reliable React Native Training course?
Several reputable online platforms, educational institutions, and technology training providers offer reliable React Native Training courses. Choosing a course that aligns with your learning goals, provides a comprehensive curriculum, and offers support from experienced instructors is essential. Some popular platforms include Udemy, Coursera, and official React Native documentation.