Looking for the Best Flutter Course to become a skilled cross-platform app developer? When building complex Flutter applications, effective state management is vital for maintaining code quality, enhancing performance, and accelerating the development process. One standout solution for such a necessity is the Bloc (Business Logic Component) Pattern.Â
Leveraging the principles of reactive programming, Bloc Pattern introduces a streamlined and efficient way of managing application state. Understanding how to properly test your Bloc implementations is also crucial. The bloc_test package is a great resource for testing Bloc Widgets, Cubits, and Blocs.
Fundamentally, the Bloc Pattern separates the business logic from the UI layer.Â
This separation endows your codebase with enhanced maintainability, fosters a cleaner structure, and makes it easier to test and modify your code.Â
Moreover, it provides a robust system to handle changes in application state, bringing a more declarative approach to state management.
The flutter_bloc package is particularly useful, as it simplifies the integration of blocs and cubits into Flutter applications
To dive into the specifics, we'll be referencing key resources including the official GitHub repository for the Bloc library, the official documentation, the Bloc package, the Bloc Test package, and the Flutter Bloc package.
In this blog post, we'll delve into the depths of Bloc Pattern, elucidating its implementation, advantages, potential challenges, and best practices, accompanied by practical examples and code snippets. Buckle up as we embark on this insightful journey of mastering the Bloc Pattern in Flutter!
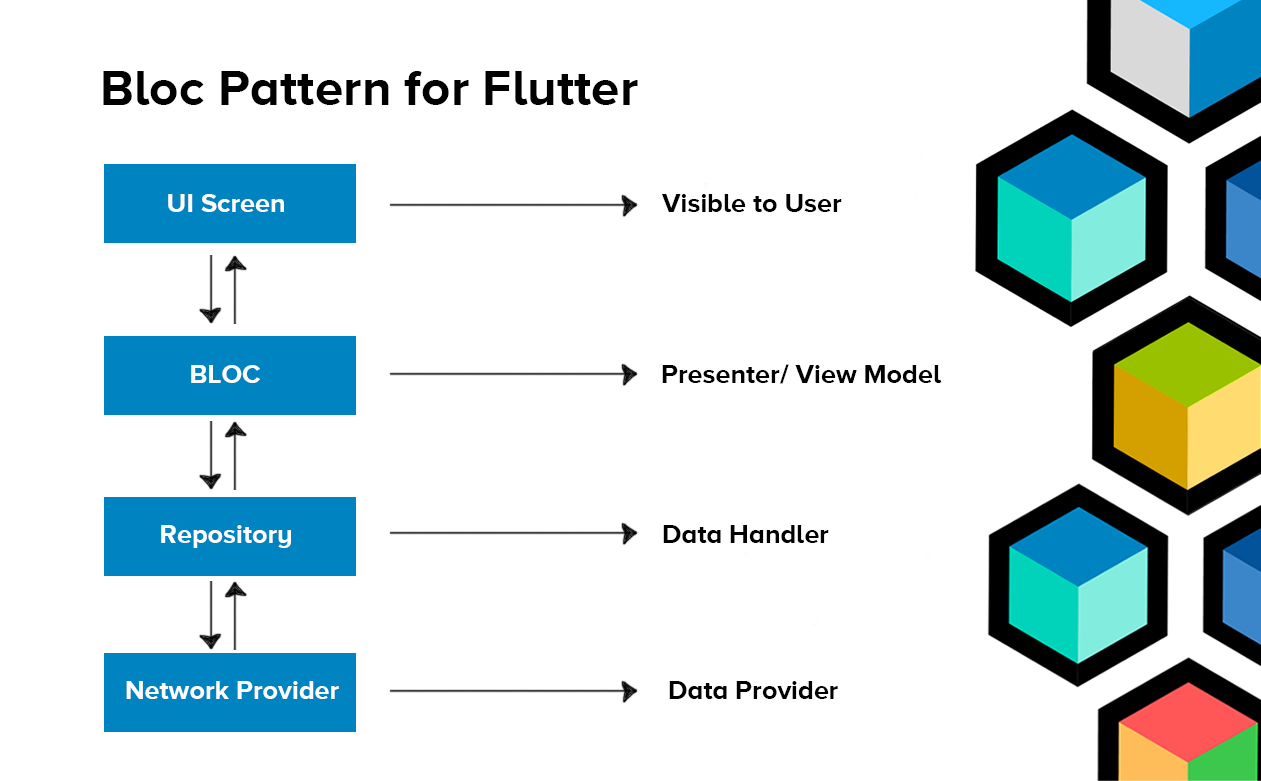
Bloc Pattern: A Deep Dive into Reactive State Management
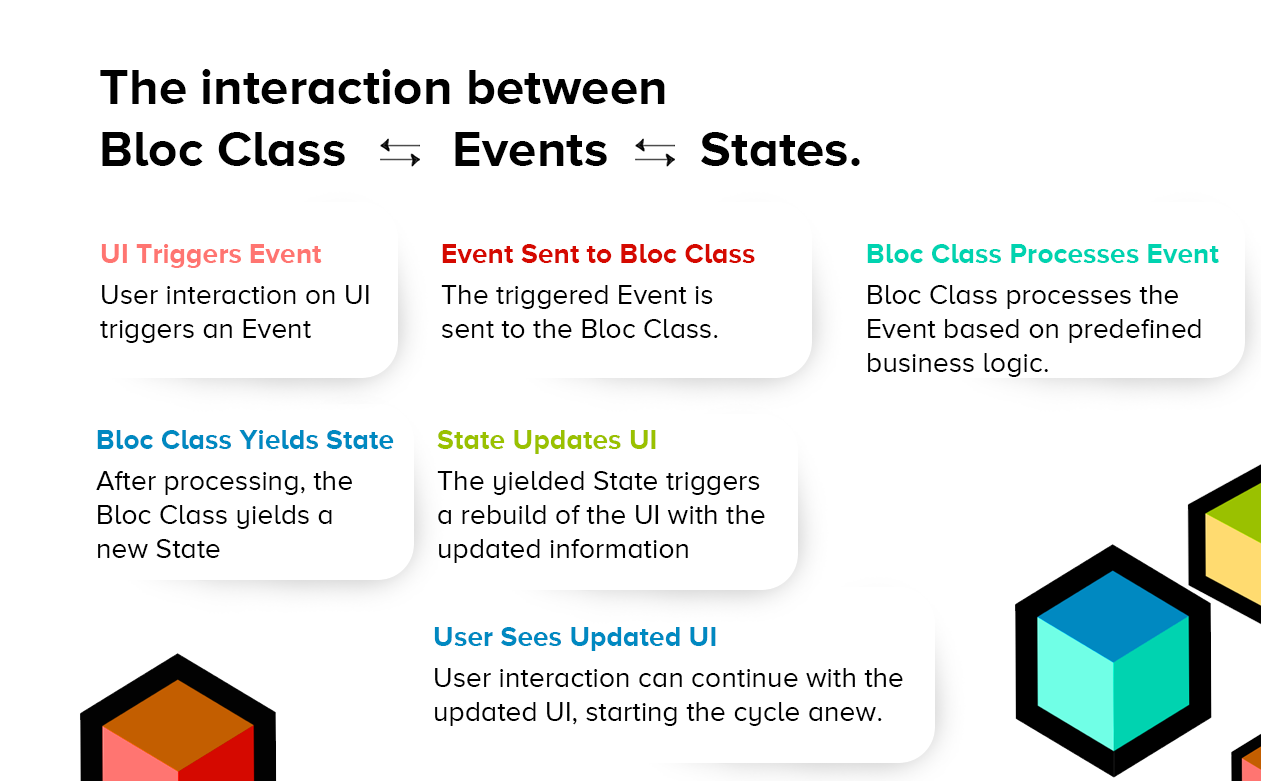
One of the foundational concepts behind the Bloc Pattern is Reactive Programming. Reactive Programming is an asynchronous programming paradigm concerned with data streams and the propagation of change. With this, you can easily propagate changes in your application state to different parts of your app, ensuring they reactively update themselves as needed.
The Bloc Pattern fits beautifully into this paradigm. It turns the state into a stream of data that components can listen to and react upon. Whenever the state changes, listeners are notified, and the UI updates automatically. This promotes a more efficient and declarative way of managing the application state.
At the core of Bloc Pattern are three primary entities:
- Bloc Class: This serves as a bridge between Events and States. It receives Events as input and maps them to new States as output.
- Events: These are inputs to a Bloc. They represent a request to transition from one state to another.
- States: These are outputs from a Bloc. They represent a part of your app's state at a particular point in time.
Now, let's delve into code and understand how to define Events and States for a simple counter application:
First, we define our Events:
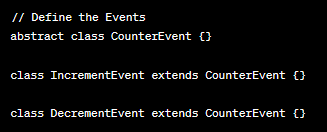
Here, IncrementEvent and DecrementEvent represent the possible events our counter can experience.
Next, we define our States:
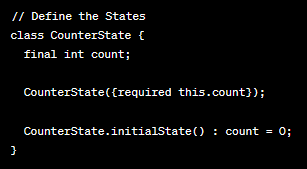
CounterState encapsulates the count value that the app needs to track. The initial state method helps in defining the initial state of our counter.
This method of defining Events and States sets a clean and easy-to-understand foundation for our Bloc Pattern implementation. The next step will be to understand the 'Bloc Class' and how to handle the transition from Events to States.
You may Enroll in a Flutter UI Design Course to know in-depth about the principles and master Flutter!
Bloc Pattern Implementation in Flutter
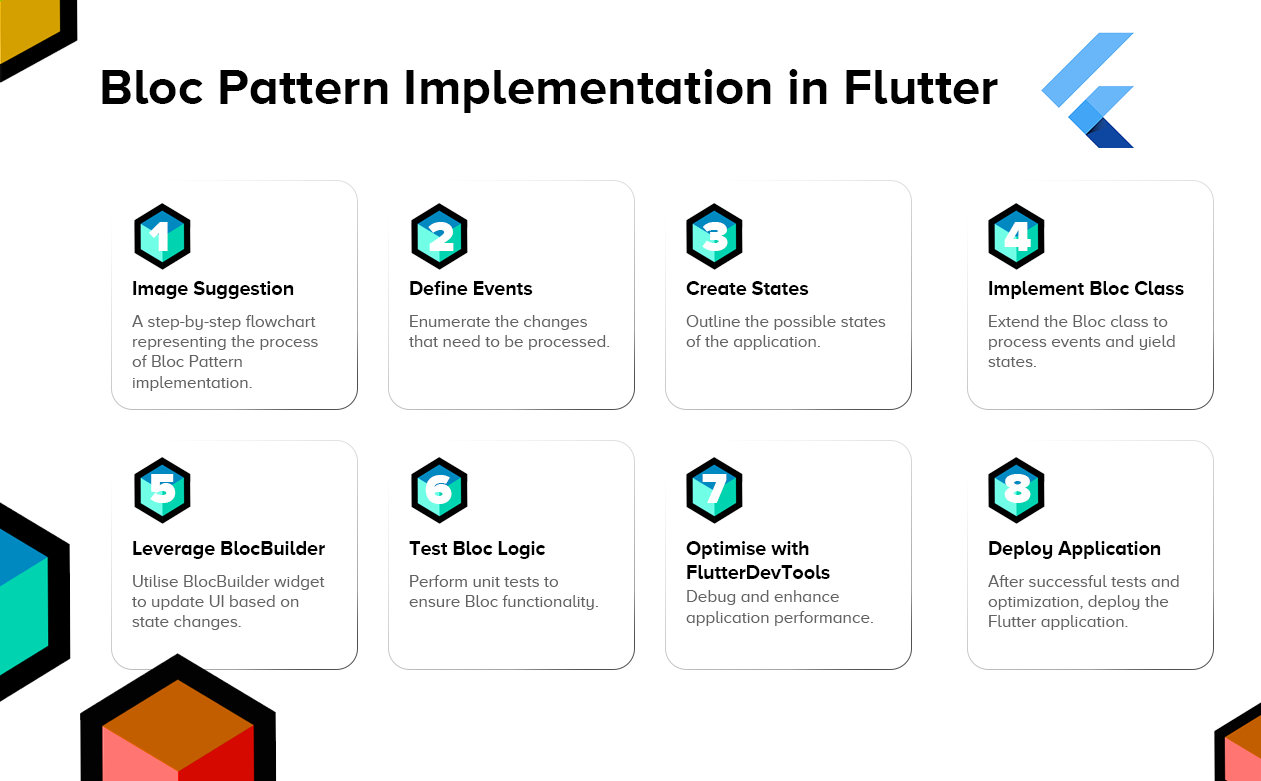
To understand how the Bloc Pattern is implemented in Flutter applications, it is necessary to install and import the bloc and flutter_bloc packages. Let's start with a step-by-step process on how to integrate these packages into our application.
Adding Dependencies:
The first step is to add these dependencies into your pubspec.yaml file:
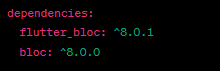
Defining Events and States:
Events are defined as classes extending from a base CounterEvent class, and states are defined similarly. Here is how you can do it:
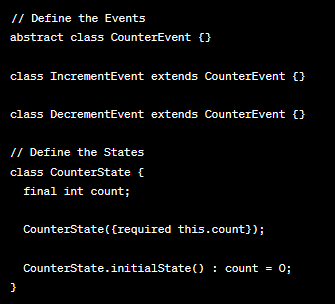
Creating the Bloc Class:
Now, we can create the CounterBloc class that will receive these events and output new states:
Using BlocBuilder to Connect UI with Bloc:
To connect our application's UI with the CounterBloc and reflect state changes, we will use the BlocBuilder widget from the flutter_bloc package. Let's create a UI that displays the current count and has buttons to trigger IncrementEvent and DecrementEvent:
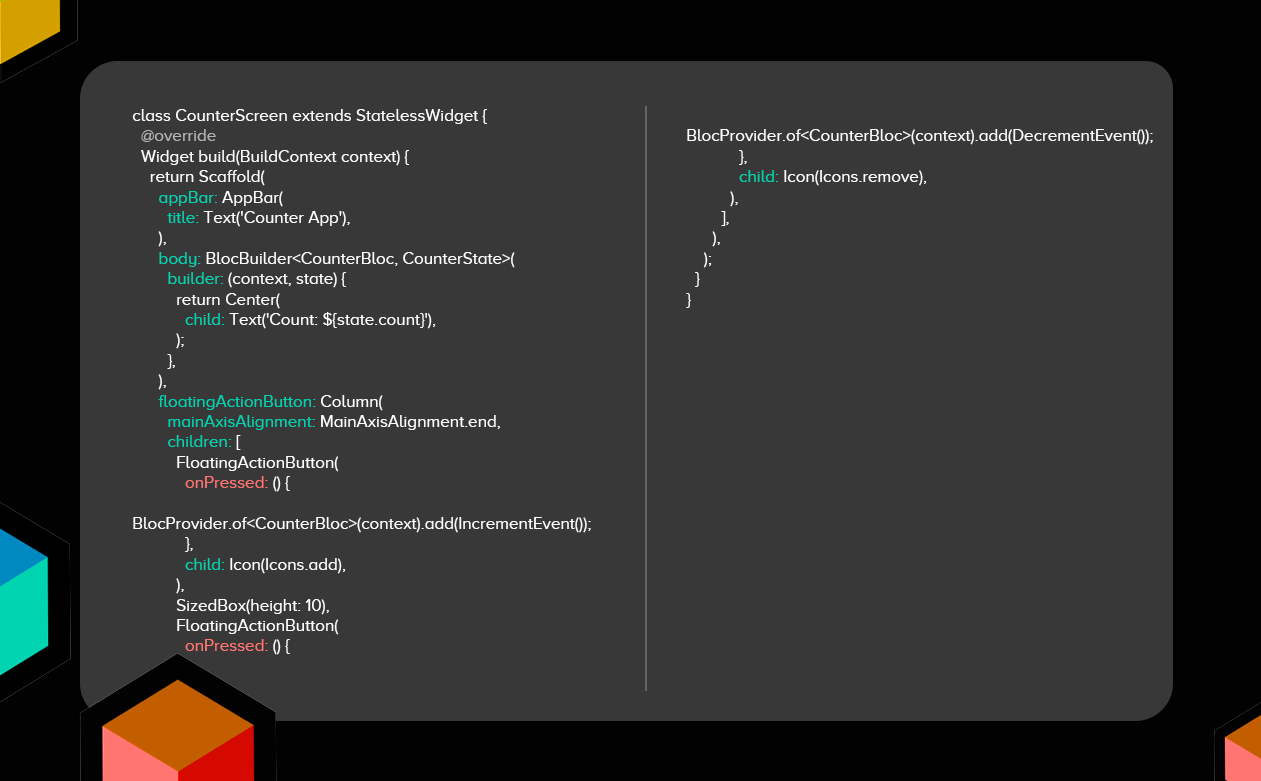
In the above CounterScreen widget, the count value is displayed in the centre of the screen, and two FloatingActionButtons are used to trigger the IncrementEvent and DeccrementEvent. Whenever an event is triggered, the UI is automatically updated to reflect the latest state, thanks to the power of the Bloc Pattern.
In order to effectively implement the Bloc pattern in Flutter, it is essential to gain a strong understanding of Android development principles and techniques. Enrolling in an Android Training Course can provide the necessary knowledge and skills to seamlessly integrate the Bloc pattern into your Flutter projects, ensuring efficient state management and improved application architecture.
The Upside: Advantages of the Bloc Pattern
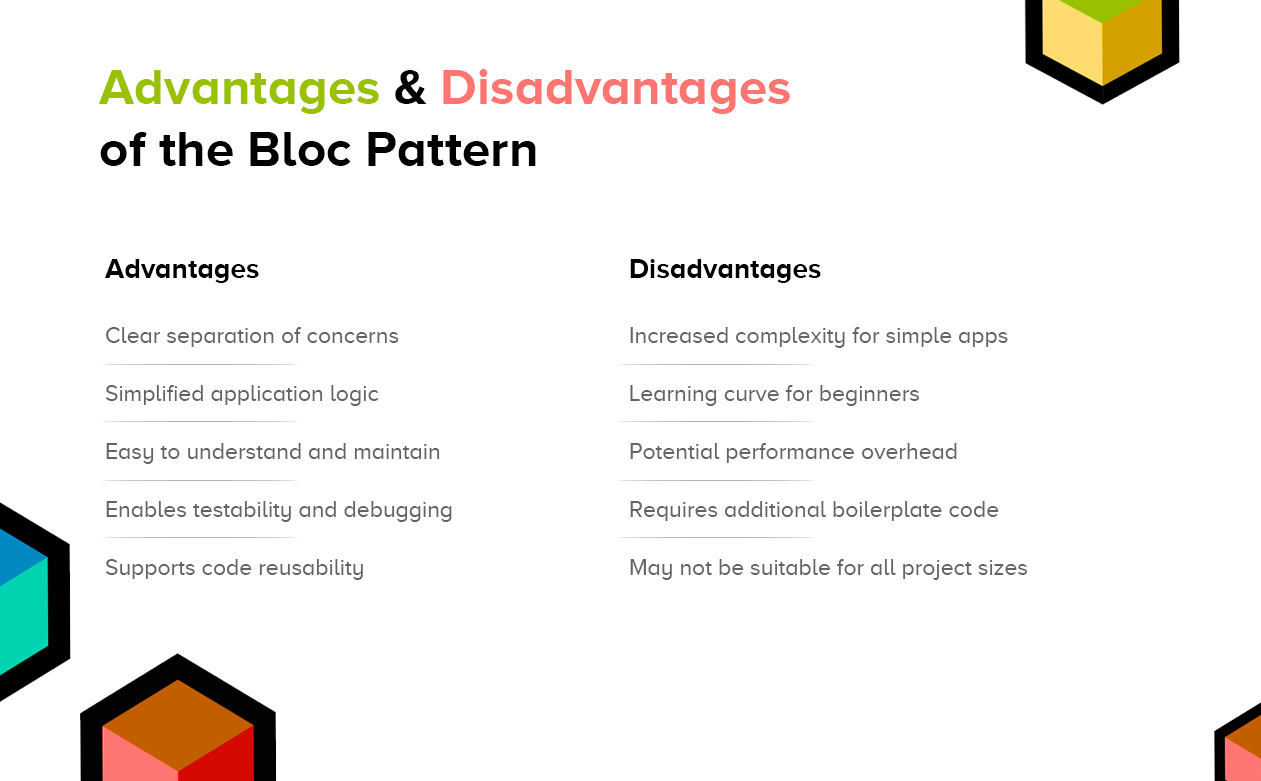
The Bloc pattern has numerous advantages that make it an excellent choice for state management in Flutter applications:
Separation of Concerns: The Bloc pattern neatly separates business logic from UI, promoting cleaner, more maintainable code. Changes to your business logic won't affect the UI layer.
Testability: The separation of business logic from UI also enhances testability. Unit tests can focus on Bloc classes without getting entangled in UI complexities.
Code Reusability: Bloc promotes code reusability by encapsulating business logic that can be reused across multiple components of the application. This approach saves time and reduces redundancy.
Improved Performance: With Bloc, UI layer updates only occur when there is a change in application state, reducing the number of re-renders and boosting app performance.
Scalability: Whether your application is small or large, the Bloc pattern scales beautifully. It effectively manages the complexity of your codebase as your application grows in size.
The Downside: Challenges with the Bloc Pattern
While the Bloc pattern offers significant advantages, developers may also encounter certain challenges:
Complexity: Implementing the Bloc pattern can add complexity to your application due to the need for additional classes and code. This added complexity may pose a learning hurdle for new developers.
Learning Curve: Developers new to Flutter or state management patterns may find the Bloc pattern challenging to master. It requires an understanding of Reactive Programming, which may not be straightforward for all developers.
Overhead: While Bloc enhances manageability and scalability, it also introduces additional code to manage the application's state, which can affect performance if not implemented correctly.
Maintenance: Compared to other state management patterns, Bloc can sometimes be more challenging to maintain. Changes to the business logic may require adjustments to multiple classes, potentially complicating maintenance efforts.
Best Practices for Effective Bloc Pattern Implementation
Focused Bloc Class
A Bloc class should be dedicated to managing a specific feature or functionality. Keeping a Bloc class small and focused ensures maintainability and easier testing.
Consider the following code snippet for a CounterBloc:
This CounterBloc is solely focused on managing the state for a counter feature.
Using the Equatable Package
The Equitable package simplifies equality comparisons. It allows you to check equality based on the object's properties, leading to easier state comparisons in Bloc.
By extending Equatable, CounterState can easily compare states based on the count property.
BlocProvider
BlocProvider helps manage the lifecycle of a Bloc and provide it to the widgets in the subtree. It ensures that only one instance of a Bloc is used throughout the application.
In this snippet, BlocProvider makes CounterBloc available to CounterPage.
BlocBuilder
BlocBuilder rebuilds the UI in response to state changes. This ensures that the UI is always synchronised with the latest state of the application.
Here, BlocBuilder rebuilds the Text widget every time CounterState changes.
FlutterDevTools
FlutterDevTools provides a suite of debugging and performance tools. It helps to visualize and understand application state and performance issues.
While FlutterDevTools doesn't involve direct coding, you can use it by running the command flutter run --start-paused in your terminal, then opening a browser to the given localhost URL. It's an indispensable tool for optimizing and debugging your Flutter apps.
Following these best practices can make your Bloc Pattern implementation more maintainable, robust, and efficient.
To truly excel in Flutter development and unlock lucrative opportunities, consider Enrolling in a
Flutter Course in Ahmedabad. By mastering Flutter's powerful UI capabilities, you'll be equipped to create visually stunning and user-friendly interfaces, setting yourself apart as a highly skilled developer.
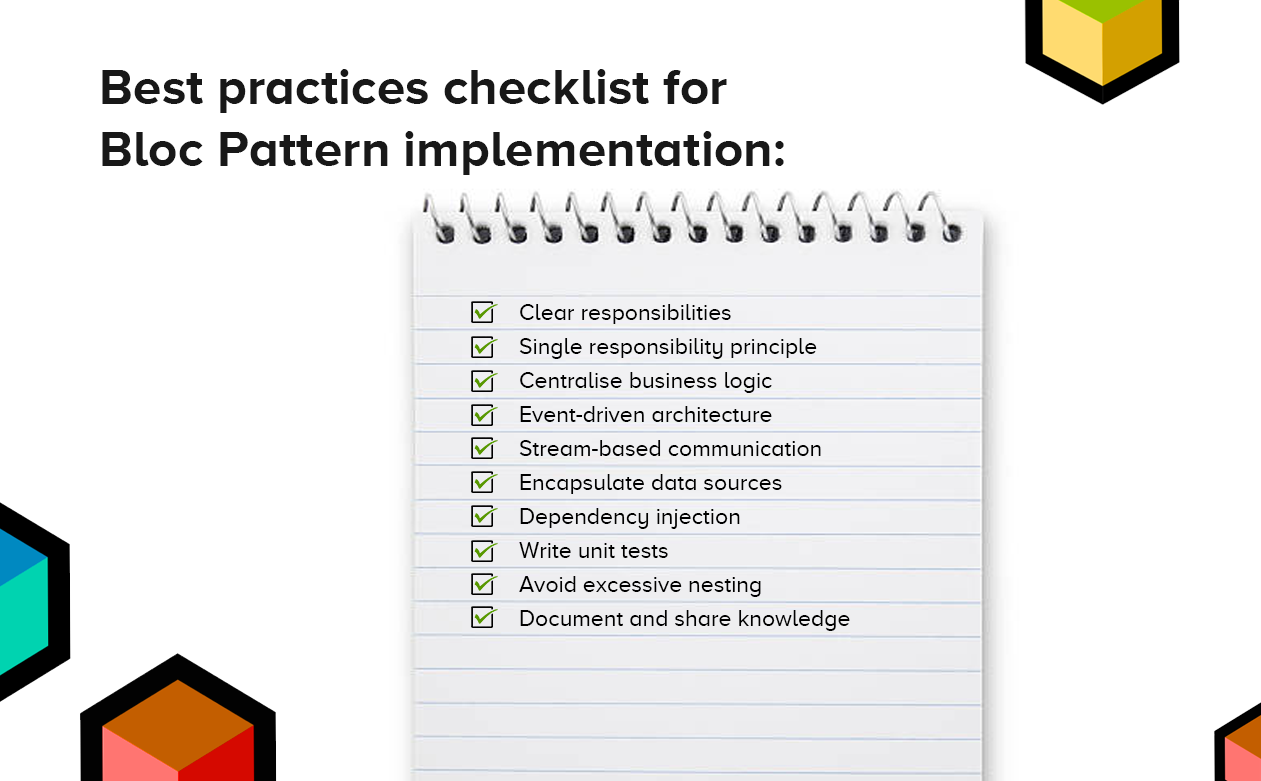
Bloc Pattern: Essential Flutter Packages
bloc
The bloc package forms the basis of the Bloc Pattern, providing essential components like Bloc, Events, and States.
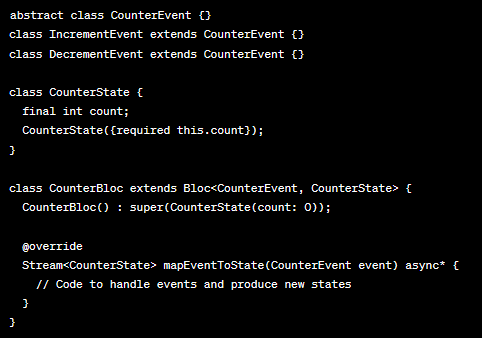
Here, we define Events and States, and create a Bloc class to handle events and produce new states.
flutter_bloc
The flutter_bloc package provides additional Flutter-specific components, such as BlocBuilder and BlocProvider.
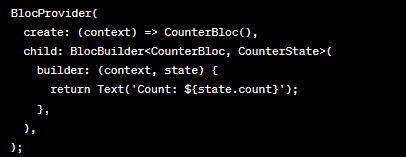
BlocProvider provides an instance of CounterBloc to the subtree, and BlocBuilder rebuilds the UI in response to state changes.
hydrated_bloc
The hydrated_bloc package allows Bloc State to be persisted and restored, ensuring the application's state survives app restarts.
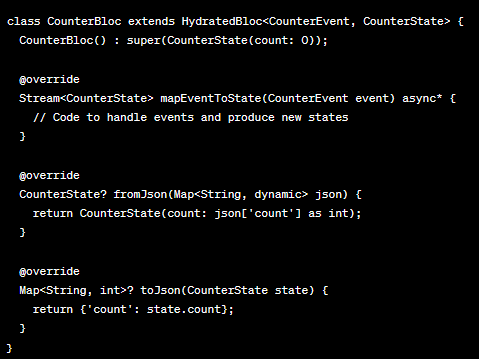
Here, CounterBloc extends HydratedBloc. The fromJson and toJson methods facilitate the saving and restoration of states.
bloc_test
The bloc_test package aids in testing the Blocs, providing utilities to easily mock and verify Bloc behaviours.
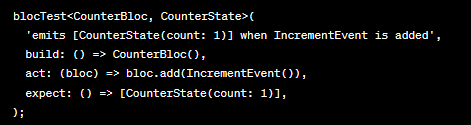
Here, blocTest is used to test that the CounterBloc correctly handles IncrementEvent and produces the expected state.
freezed
The freezed package aids in generating immutable classes, reducing boilerplate code and making states and events easier to define and manage.
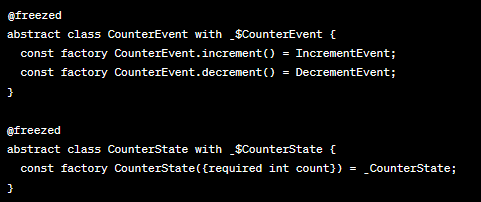
Here, freezed generates code for CounterEvent and CounterState,
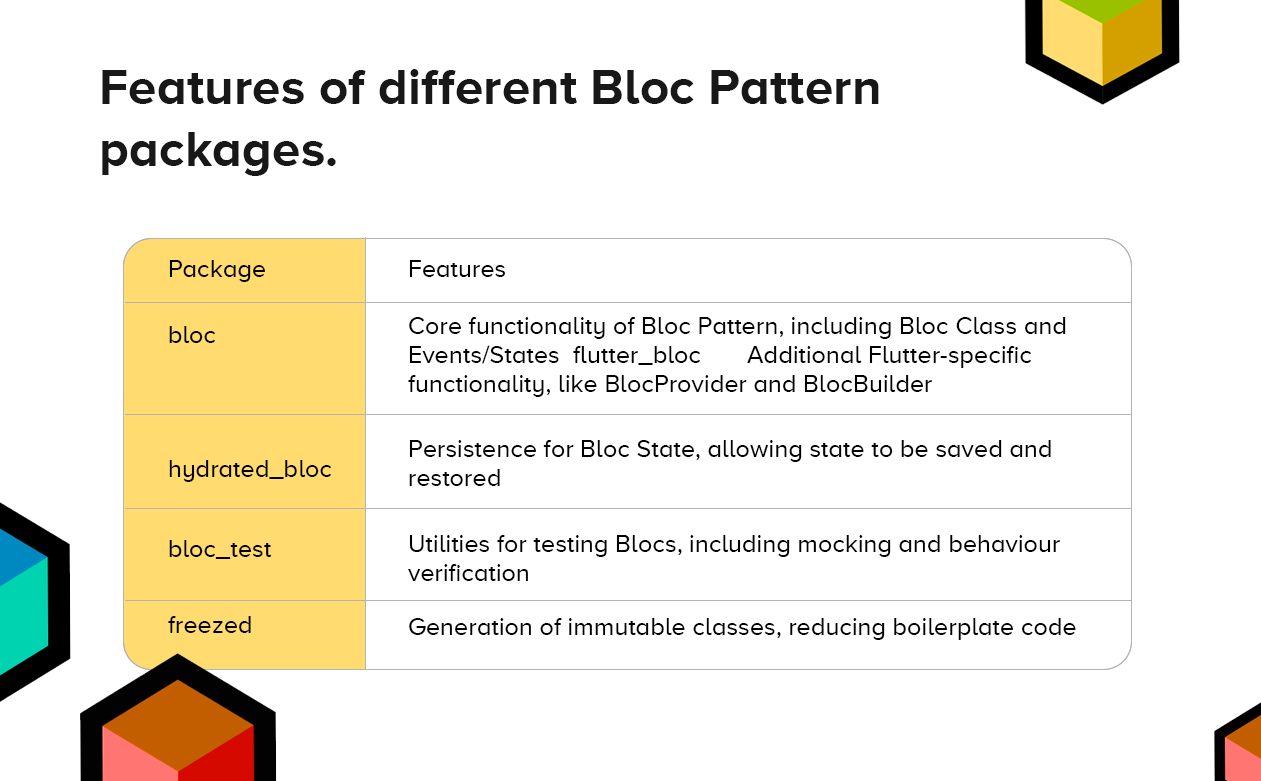
Enroll In The Best Flutter Course!
When it comes to learning Flutter,
TOPS Technologies stands out as the premier destination. With an exceptional track record and an extensive range of courses, TOPS Technologies has been a trusted name in the IT Training & Placement industry for over 15 years. Having successfully placed over 1 lakh students, we have solidified our reputation for delivering quality training and creating promising career opportunities.
With 19 offices spread across India, TOPS Technologies
ensures convenience and accessibility for learners in multiple locations. Our portfolio boasts over 50 industry courses, including
iOS Certification Courses,
Android training courses, etc. showcasing our versatility and commitment to meeting diverse learning needs.
By choosing TOPS Technologies for Flutter training, students gain access to our wealth of experience, strong industry connections, and a comprehensive range of courses. Students will be taught Flutter from the basics like
what is flutter, basic UI , etc all the way up to the advanced level. This sets them up for success in the ever-evolving field of mobile app development.
Conclusion
The Bloc Pattern in Flutter has revolutionised the way developers manage application state, providing a powerful tool for crafting efficient, scalable, and maintainable apps. By focusing on states and events, Bloc promotes a structured, logical approach to app development. However, as with any tool, mastery comes with understanding and practising the best methodologies.
That's where a comprehensive course like the one at TOPS Technologies shines, providing an in-depth understanding of Flutter and Bloc from the basics to advanced topics. By enrolling in our
Flutter course in Surat you can craft impressive Flutter applications using Bloc, navigating the exciting world of mobile app development with confidence and proficiency.
Remember, as Walt Disney said, "The way to get started is to quit talking and start doing."
FAQs
What is the Bloc Pattern in Flutter?
The Bloc (Business Logic Component) Pattern is a state management system in Flutter that uses reactive programming principles. It separates the business logic from the UI, making applications easier to maintain and test.
How does the Bloc Pattern compare to other state management systems like Provider or Redux?
While Bloc, Provider, and Redux are all state management systems, Bloc's event-driven nature often makes it easier to manage complex states. It's also highly testable and promotes a clear separation between business logic and the UI.
How can I implement the Bloc Pattern in Flutter?
To implement the Bloc Pattern, you'll need the bloc and flutter_bloc packages. You define Events and States, create a Bloc class that extends the Bloc package's Bloc class, and then use the BlocBuilder widget to update the UI based on state changes.
What are the advantages of using the Bloc Pattern in Flutter?
Bloc Pattern provides several benefits, such as separation of concerns, improved testability, code reusability, and increased performance. It's also scalable and can help manage code complexity in larger applications.
What are some best practices when using the Bloc Pattern in Flutter?
Best practices include keeping the Bloc Class focused, using packages like Equatable for simpler state implementation, leveraging BlocProvider for providing Bloc instances to the UI, and using FlutterDevTools for debugging and performance optimization.